Java Integration
Transform Anything with Java
Differenece between portType and Opeartion
Let us create a service with 2 port type.
1. Let us create a xsd that gets 2 strings and returns its in reverse and straight concatination. This can be done in any editor and I did it in notepad++ and named it StringOperation.xsd
<?xml version= '1.0' encoding= 'UTF-8' ?>
<schema attributeFormDefault="unqualified" elementFormDefault="qualified" targetNamespace="http://example.org/string/types/"
xmlns:tns="http://example.org/string/types/" xmlns="http://www.w3.org/2001/XMLSchema">
<complexType name="StringInput">
<sequence>
<element name="x" type="string"/>
<element name="y" type="string"/>
</sequence>
</complexType>
<complexType name="StringOutput">
<sequence>
<element name="result" type="string"/>
</sequence>
</complexType>
<element name="StraightConcat" type="tns:StringInput"/>
<element name="StraightConcatResponse" type="tns:StringOutput"/>
<element name="ReverseConcat" type="tns:StringInput"/>
<element name="ReverseConcatResponse" type="tns:StringOutput"/>
</schema>
3. Open JDeveloper > File > New > SOA Project > Name the project as Stringtwoport > Composite with BPEL Process
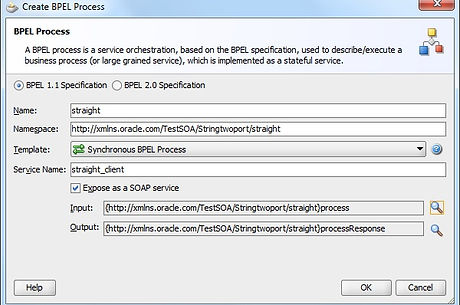
4. In the input and output, choose the xsd that we created and choose StraightConcat and StraightConcatResponse
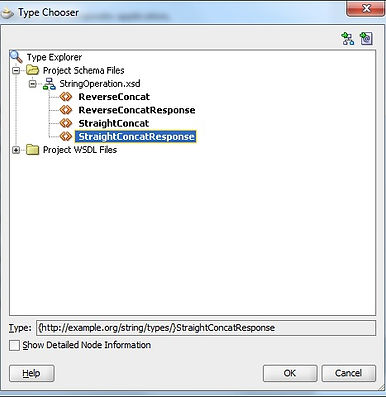
5. Add another BPEL Component to the Composite. Name it as reverse.
6. Choose ReverseConcat and ReverseConcatResponse as input and output. Your composite should look like below.
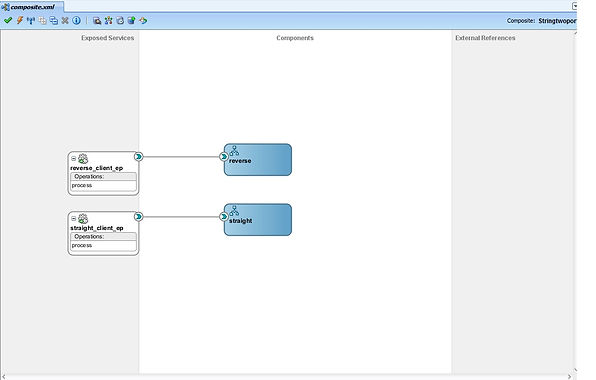
The implementation can be by any means, but since there are 2 BPEL components, the composite will be exposed with 2 endpoints like below
straight_client_ep and reverse_client_ep.
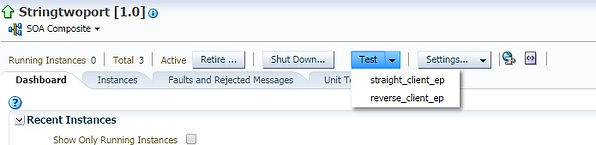
Now let us create a SOA application with 2 operation. This is the ideal way of exposing SOA application to the real world.
1. Create a new SOA Project with an empty composite. The input and output should be same as the previous example. In this case, just select StraightInput and StraightOutput
2. Open the wsdl and add the item which is in bold
<wsdl:portType name="BPELProcess1">
<wsdl:operation name="straight">
<wsdl:input message="client:BPELProcess1RequestMessage" />
<wsdl:output message="client:BPELProcess1ResponseMessage"/>
</wsdl:operation>
<wsdl:operation name="reverse">
<wsdl:input message="client:BPELProcess1RequestMessage" />
<wsdl:output message="client:BPELProcess1ResponseMessage"/>
</wsdl:operation>
</wsdl:portType>
This means that you are adding an extra operation to the portTypeBPELProcess1.
3. Here in this case we cannot use 2 BPEL composite, instead we are going to remove the receive activity from the BPEL and use a Pick activity like below
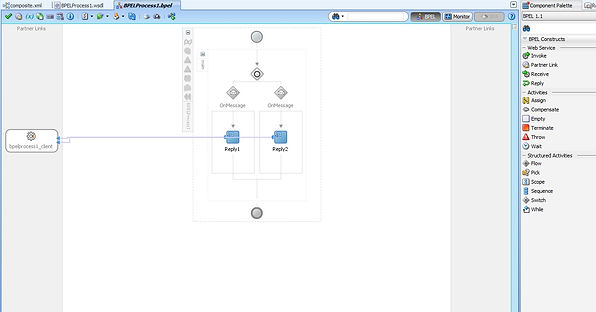
4. Attach the reply activity from both the OnMessage and select the partner Link and operation straight and Reverse for each one.
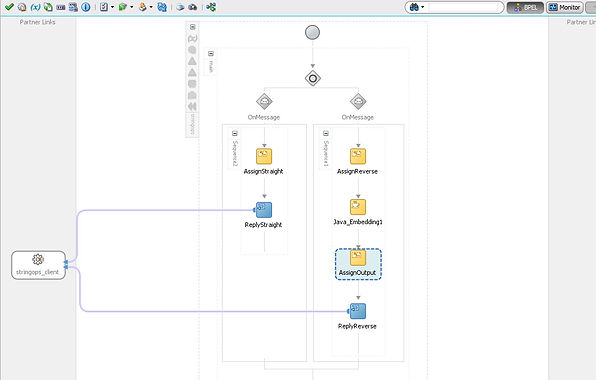
The BPEL composite should be like above.
Note : The pick activity should have "Create Instance" property checked.
Below is the code that I used for Java Embedding to reverse String. Very simple code, but there are many ways a string reverse can be done.
String original1, reverse1 = "";
String original2, reverse2 = "";
original1 = (String)getVariableData("inputString1");
original2 = (String)getVariableData("inputString2");
System.out.println("Original1"+original1);
System.out.println("Original2"+original2);
int len = original1.length();
for (int i=len-1; i>=0; i--)
reverse1=reverse1+original1.charAt(i);
len = original2.length();
for (int i=len-1; i>=0; i--)
reverse2=reverse2+original2.charAt(i);
System.out.println("reverse1"+reverse1);
System.out.println("reverse2"+reverse2);
setVariableData("outputString1", reverse1);
setVariableData("outputString2", reverse2);
Now let us look at the output of the service.
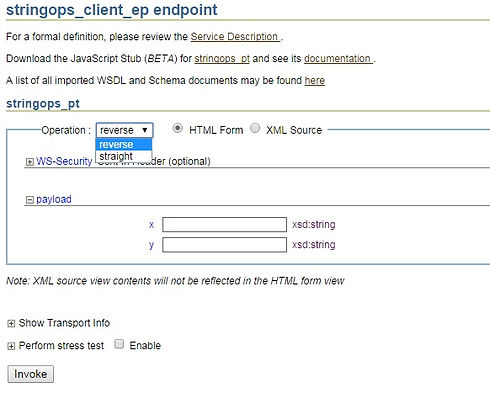
