Java Integration
Transform Anything with Java
1. To demonstrate, let me deploy 2 helloworld SOA composite and take the URL.
2. We will also create a DVM that will contain a mapping of URL and entry String. (1 for helloworld1 and 2 for helloworld2)
http://localhost:7001/soa-infra/services/poc/HelloWorld!1.0*soa_5fd3eea6-de80-4429-91dc-092b0b505dc2/helloworld_client_ep
http://localhost:7001/soa-infra/services/poc/HelloWorld/helloworld_client_ep
The below URL has details of working with DVM.
http://docs.oracle.com/cd/E14571_01/integration.1111/e10224/med_dvm.htm
3. To create a DVM, you can use JDeveloper as below
Oracle SOA simple MDS/DVM with Dynamic PartnerLink
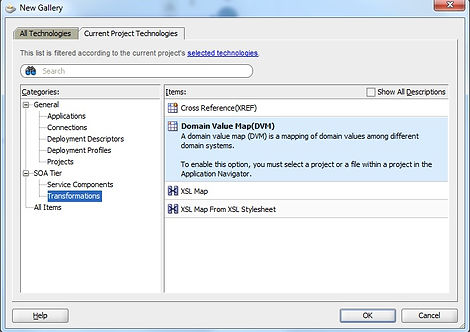
The DVM Should look as below
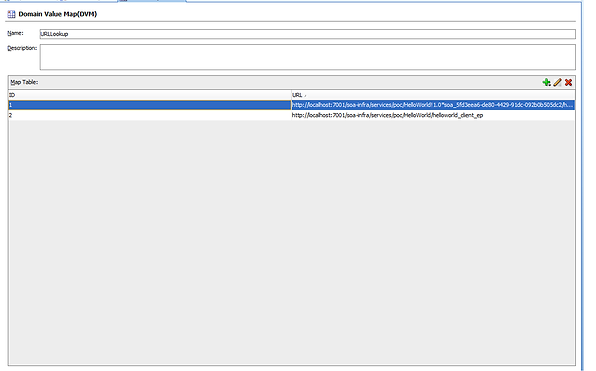
The Source for DMV will look like below
<?xml version="1.0" encoding="UTF-8" ?>
<!-- Generated by Oracle DVM Editor version 1.0 at [9/8/14 10:46 AM]. -->
<dvm name="URLLookup" xmlns="http://xmlns.oracle.com/dvm">
<description>
</description>
<columns>
<column name="ID" qualifier="true"/>
<column name="URL"/>
</columns>
<rows>
<row>
<cell>1</cell>
<cell>http://localhost:7001/soa-infra/services/poc/HelloWorld!1.0*soa_5fd3eea6-de80-4429-91dc-092b0b505dc2/helloworld_client_ep</cell>
</row>
<row>
<cell>value</cell>
<cell>http://localhost:7001/soa-infra/services/poc/HelloWorld/helloworld_client_ep</cell>
</row>
</rows>
</dvm>
To deploy the DVM, place the dvm file insite folders apps/dvms
zip the apps folder to apps.zip.
Open EM Console > soa-infra > Administration > MDS Configuration
Import the apps.zip.
Validate the DVM with the below URL.
http://localhost:7001/soa-infra/services/poc/HelloWorld/apps/dvms/URLLookup.dvm
4. Create a SOA project with emplty BPEL Process. Let the service be synchronous.
5. Since we are going to route dynamically, we will add ws-addressing.xsd to the wsdl.
<xsd:schema xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<xsd:import namespace="http://schemas.xmlsoap.org/ws/2003/03/addressing" schemaLocation="oramds:/soa/shared/common/ws-addressing.xsd"/>
</xsd:schema>
Below is the code for BPEL to dynamically assign the endpoint
<assign name="AssignURL">
<copy>
<from expression="dvm:lookupValue('oramds:/apps/dvms/URLLookup.dvm','ID',bpws:getVariableData('inputVariable','payload','/client:process/client:ID'),'ServiceEndPoint','NoData')"/>
<to variable="endPointReference"
query="/ns2:EndpointReference/ns2:Address"/>
</copy>
<copy>
<from variable="endPointReference"/>
<to partnerLink="helloworld"/>
</copy>
</assign>
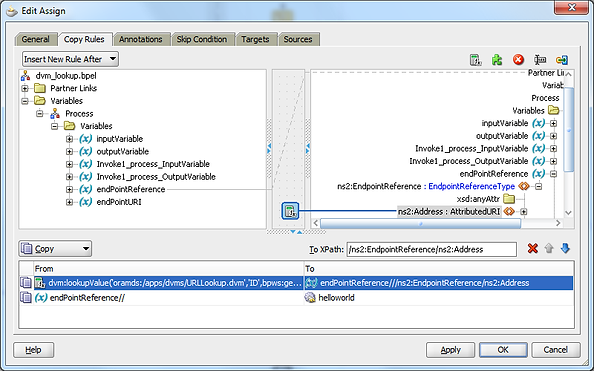
<?xml version = "1.0" encoding = "UTF-8" ?>
<!--
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
Oracle JDeveloper BPEL Designer
Created: Mon Sep 08 13:44:10 PDT 2014
Author: bala
Type: BPEL 1.1 Process
Purpose: Synchronous BPEL Process
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
-->
<process name="dvm_lookup"
targetNamespace="http://xmlns.oracle.com/POC/DVM_Lookup/dvm_lookup"
xmlns="http://schemas.xmlsoap.org/ws/2003/03/business-process/"
xmlns:client="http://xmlns.oracle.com/POC/DVM_Lookup/dvm_lookup"
xmlns:ora="http://schemas.oracle.com/xpath/extension"
xmlns:bpelx="http://schemas.oracle.com/bpel/extension"
xmlns:bpws="http://schemas.xmlsoap.org/ws/2003/03/business-process/"
xmlns:ns1="http://xmlns.oracle.com/POC/HelloWorld/helloWorld"
xmlns:ns2="http://schemas.xmlsoap.org/ws/2003/03/addressing"
xmlns:bpel2="http://docs.oasis-open.org/wsbpel/2.0/process/executable"
xmlns:xp20="http://www.oracle.com/XSL/Transform/java/oracle.tip.pc.services.functions.Xpath20"
xmlns:oraext="http://www.oracle.com/XSL/Transform/java/oracle.tip.pc.services.functions.ExtFunc"
xmlns:dvm="http://www.oracle.com/XSL/Transform/java/oracle.tip.dvm.LookupValue"
xmlns:hwf="http://xmlns.oracle.com/bpel/workflow/xpath"
xmlns:ids="http://xmlns.oracle.com/bpel/services/IdentityService/xpath"
xmlns:bpm="http://xmlns.oracle.com/bpmn20/extensions"
xmlns:xdk="http://schemas.oracle.com/bpel/extension/xpath/function/xdk"
xmlns:xref="http://www.oracle.com/XSL/Transform/java/oracle.tip.xref.xpath.XRefXPathFunctions"
xmlns:ldap="http://schemas.oracle.com/xpath/extension/ldap"
xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<!--
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
PARTNERLINKS
List of services participating in this BPEL process
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
-->
<partnerLinks>
<!--
The 'client' role represents the requester of this service. It is
used for callback. The location and correlation information associated
with the client role are automatically set using WS-Addressing.
-->
<partnerLink name="dvm_lookup_client" partnerLinkType="client:dvm_lookup" myRole="dvm_lookupProvider"/>
<partnerLink name="helloworld" partnerLinkType="ns1:helloWorld"
partnerRole="helloWorldProvider"/>
</partnerLinks>
<!--
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
VARIABLES
List of messages and XML documents used within this BPEL process
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
-->
<variables>
<!-- Reference to the message passed as input during initiation -->
<variable name="inputVariable" messageType="client:dvm_lookupRequestMessage"/>
<!-- Reference to the message that will be returned to the requester-->
<variable name="outputVariable" messageType="client:dvm_lookupResponseMessage"/>
<variable name="Invoke1_process_InputVariable"
messageType="ns1:helloWorldRequestMessage"/>
<variable name="Invoke1_process_OutputVariable"
messageType="ns1:helloWorldResponseMessage"/>
<variable name="endPointReference" element="ns2:EndpointReference"/>
<variable name="endPointURI" type="xsd:string"/>
</variables>
<!--
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
ORCHESTRATION LOGIC
Set of activities coordinating the flow of messages across the
services integrated within this business process
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
-->
<sequence name="main">
<!-- Receive input from requestor. (Note: This maps to operation defined in dvm_lookup.wsdl) -->
<receive name="receiveInput" partnerLink="dvm_lookup_client" portType="client:dvm_lookup" operation="process" variable="inputVariable" createInstance="yes"/>
<!-- Generate reply to synchronous request -->
<assign name="Assign1">
<copy>
<from expression="dvm:lookupValue('oramds:/apps/dvms/URLLookup.dvm','ID',bpws:getVariableData('inputVariable','payload','/client:process/client:ID'),'ServiceEndPoint','NoData')"/>
<to variable="endPointURI"/>
</copy>
</assign>
<assign name="AssignURL">
<copy>
<from expression="dvm:lookupValue('oramds:/apps/dvms/URLLookup.dvm','ID',bpws:getVariableData('inputVariable','payload','/client:process/client:ID'),'ServiceEndPoint','NoData')"/>
<to variable="endPointReference"
query="/ns2:EndpointReference/ns2:Address"/>
</copy>
<copy>
<from variable="endPointReference"/>
<to partnerLink="helloworld"/>
</copy>
</assign>
<bpelx:exec name="Java_Embedding1" version="1.5" language="java">
<![CDATA[System.out.println("Address in "+getVariableData("endPointURI"));]]>
</bpelx:exec>
<invoke name="invoke_end"
inputVariable="Invoke1_process_InputVariable"
outputVariable="Invoke1_process_OutputVariable"
partnerLink="helloworld" portType="ns1:helloWorld"
operation="process" bpelx:invokeAsDetail="no">
<bpelx:inputProperty name="wsa.to" variable="endPointURI"/>
</invoke>
<reply name="replyOutput" partnerLink="dvm_lookup_client" portType="client:dvm_lookup" operation="process" variable="outputVariable"/>
</sequence>
</process>
Once this is done, deploy the composite and test with argument 1 and 2. BPEL will lookup DVM and dynamically get URL and hit the service.